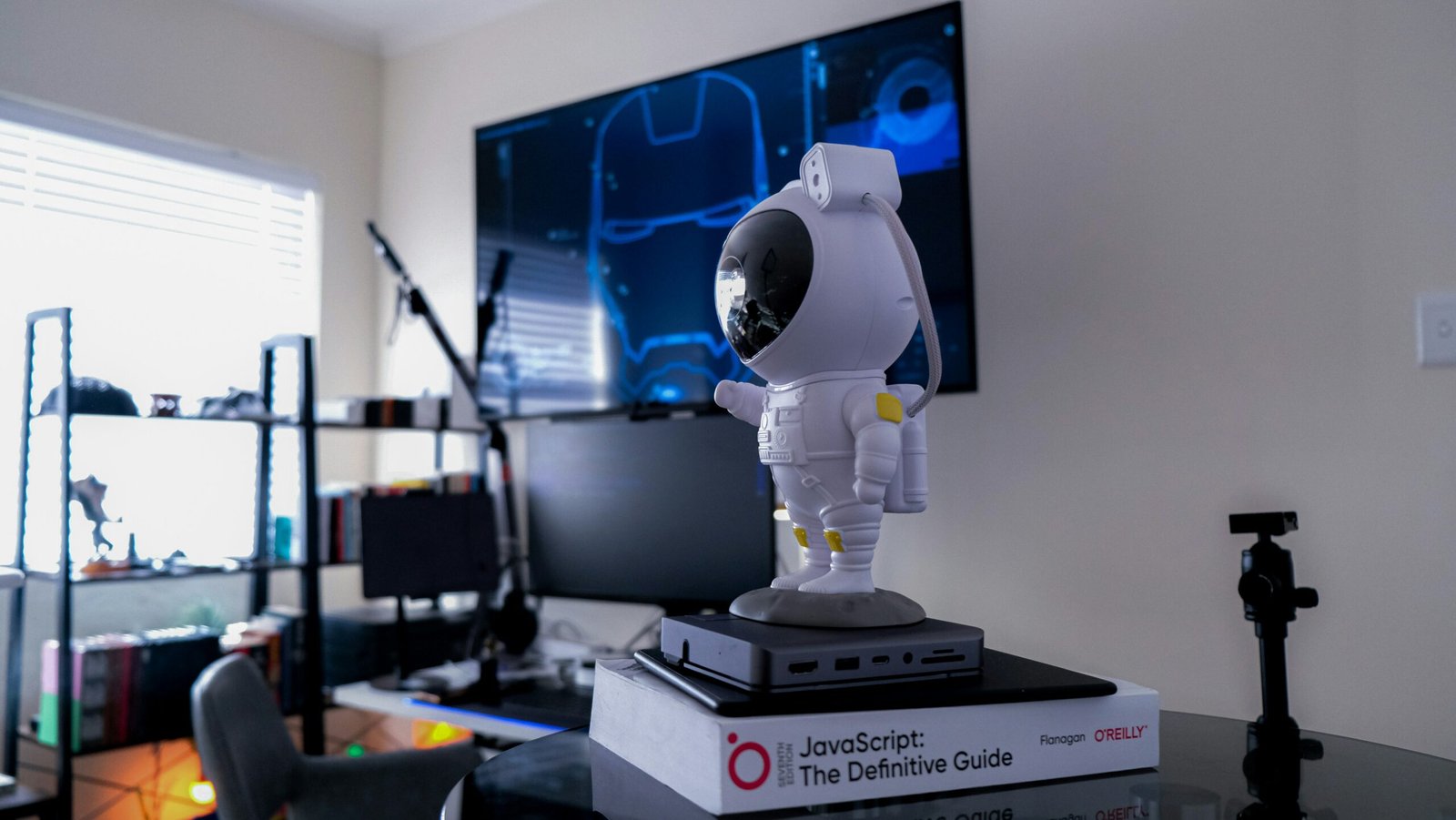
Introduction to JavaScript
JavaScript is a cornerstone of modern web development, celebrated for its ability to create dynamic and interactive user experiences. Introduced by developer Brendan Eich in 1995, JavaScript quickly established itself as an essential tool in the web development toolkit. Conceived at Netscape, the language was initially designed to enhance web pages by adding interactivity and behavior. Since its debut in the mid-1990s, JavaScript has evolved significantly, growing from a simple scripting tool to a robust and versatile programming language.
Understanding where JavaScript fits in the ecosystem of web technologies is crucial. The triad of HTML, CSS, and JavaScript forms the backbone of web development. HTML (HyperText Markup Language) primarily structures and presents content on the web. CSS (Cascading Style Sheets) handles the design and layout aspects of web pages, ensuring they are visually pleasing. JavaScript, on the other hand, empowers developers to add interactivity to their sites. It responds to user actions such as clicks, form submissions, and other events, enabling functionalities like dynamic content updates, animations, and even complex web applications.
JavaScript’s significance extends beyond mere interactivity. It underpins several transformations in web development, contributing to the rise of rich internet applications (RIAs) and single-page applications (SPAs). These applications deliver smoother, more immersive user experiences by reducing the need for full-page reloads. The language’s continual advancements, fueled by an active community and the development of powerful frameworks and libraries such as React, Angular, and Vue.js, have cemented its role in both client-side and server-side development through platforms like Node.js.
JavaScript’s adaptability and its profound impact on how web content is developed and consumed mark its importance in the contemporary digital landscape. Its journey from a supplementary scripting language to a formidable force in programming underscores its evolutionary prowess and continued relevance in the ever-evolving field of web development.
Core Concepts and Syntax
JavaScript is an essential programming language for the web, providing the functionality necessary to create dynamic and interactive user experiences. To unlock its potential, understanding its core concepts and syntax is essential. Let’s delve into the foundational elements of JavaScript.
The concept of variables in JavaScript forms the bedrock of the language. Variables are containers for storing data values and are declared using the var
, let
, or const
keywords. For example, let message = 'Hello, World!';
initializes a variable named message with the string value ‘Hello, World!’. The choice of keyword affects the scope and mutability of the variable.
JavaScript supports various data types, including primitive types such as string, number, boolean, null, undefined, and symbol as well as more complex types like objects and arrays. An understanding of these data types is crucial for data manipulation and control in JavaScript applications.
Operators in JavaScript allow you to perform computations and operations on data. These include arithmetic operators like +
, -
, *
, and /
, as well as comparison operators such as ==
, ===
, !=
, and !==
. Logical operators, like &&
(AND), ||
(OR), and !
(NOT), play a significant role in control flow decisions.
Functions are reusable blocks of code designed to perform a particular task. They are defined using the function
keyword, followed by a name and a set of parentheses that may include parameters. For instance, function greet(name) { return 'Hello ' + name; }
defines a function greet that returns a greeting message.
Control structures such as loops and conditionals direct the flow of program execution in JavaScript. If and else statements handle decision-making, evaluating whether certain conditions are met. Loops, like for
and while
, enable repetitive execution of code blocks until specific conditions are satisfied.
Understanding how JavaScript code is executed within web browsers is important. JavaScript engines, embedded within browsers, interpret and run the code. This allows web applications to become dynamic, updating content and responding to user interactions seamlessly.
Mastering these core concepts and syntax elements of JavaScript is fundamental to becoming an efficient programmer. With a solid grasp of variables, data types, operators, functions, and control structures, you can build robust and dynamic web applications.
Understanding the Document Object Model (DOM)
The Document Object Model (DOM) serves as a crucial bridge between JavaScript and HTML, forming the foundation of dynamic web content. Essentially, the DOM represents the structure of an HTML document as a tree of nodes, each corresponding to elements, attributes, or pieces of text within the document. This hierarchical model allows JavaScript to traverse, access, and manipulate the web page’s structure and styles programmatically.
JavaScript interacts with the DOM to enhance user interfaces, modify content dynamically, and respond to user inputs. For instance, when a web page is loaded, the browser parses the HTML and constructs a DOM tree, which JavaScript can subsequently access to make changes on-the-fly. This interaction is fundamental to modern web development, enabling rich, interactive user experiences.
One of the core operations in the DOM is selecting elements. Developers can utilize methods like document.getElementById()
, document.getElementsByClassName()
, and document.querySelector()
to pinpoint specific elements within the DOM tree. For instance, to select a paragraph element with an ID of “intro”, one would use:
let introParagraph = document.getElementById('intro');
Once an element is selected, JavaScript can modify its attributes and content. For example, changing the text content of the selected paragraph can be done through:
introParagraph.textContent = 'Updated Text';
Besides content modifications, JavaScript enables dynamic styling by manipulating the CSS properties of DOM elements. Using the style
object, developers can change the appearance of elements directly:
introParagraph.style.color = 'blue';
Handling user events is another powerful capability provided by the DOM. Using event listeners, JavaScript can respond to actions like clicks, key presses, and mouse movements. For example, setting up an event listener to change the text color of a paragraph when it’s clicked involves:
introParagraph.addEventListener('click', function() { this.style.color = 'red';});
In summary, the DOM is an indispensable aspect of JavaScript programming. It allows developers to interact with and manipulate the structure and style of web pages in real-time, fostering dynamic, interactive web applications. Understanding the DOM’s concept and operations is essential for any aspiring web developer aiming to harness the full potential of JavaScript.
JavaScript in Action: Examples and Use Cases
JavaScript is a powerful tool for creating dynamic and interactive web pages. Its versatility extends to a myriad of functionalities that improve user experience. This section will explore some practical examples and real-world use cases of JavaScript, showcasing its capabilities with code snippets and explanations.
Form Validation
Form validation is a common use of JavaScript, ensuring that users provide the necessary data in the correct format before submitting a form. Here is an example of client-side form validation:
document.getElementById('myForm').addEventListener('submit', function(event) { var name = document.getElementById('name').value; var email = document.getElementById('email').value; if (name === '' || email === '') { alert('All fields are required.'); event.preventDefault(); }});
In this simple form validation script, JavaScript checks if the ‘name’ and ’email’ fields are filled out. If either is empty, a warning message is displayed, and the form submission is prevented, ensuring data integrity.
Dynamic Content Updates
JavaScript also excels in updating content dynamically without the need to reload the page. For instance, consider the following example:
document.getElementById('updateButton').addEventListener('click', function() { document.getElementById('content').innerHTML = 'Content has been updated!';});
With this script, clicking a button with the ID ‘updateButton’ changes the content of an element with the ID ‘content’ to the specified text, providing a more interactive and engaging user experience.
Event Handling
Handling user events, such as clicks or hovers, is another practical application of JavaScript. This is a basic example of event handling:
document.getElementById('myButton').addEventListener('click', function() { alert('Button was clicked!');});
By attaching an event listener to the button with the ID ‘myButton’, this script displays an alert when the button is clicked, allowing for responsive user interaction.
Asynchronous Operations with AJAX
Asynchronous operations are crucial for modern web applications. AJAX (Asynchronous JavaScript and XML) enables background server communication without disrupting the user experience:
var xhr = new XMLHttpRequest();xhr.open('GET', 'data.json', true);xhr.onload = function() { if (xhr.status === 200) { var data = JSON.parse(xhr.responseText); document.getElementById('output').innerHTML = 'Data loaded: ' + data.message; }};xhr.send();
This AJAX example retrieves data from ‘data.json’ and updates the content of an element with the ID ‘output’ once the data is successfully loaded. Using AJAX for such operations keeps the user interface smooth and responsive.
Fetch API for Asynchronous Fetching
Another modern approach to handle asynchronous operations is the Fetch API. Here’s how it works:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { document.getElementById('output').innerHTML = 'Fetched Data: ' + data.message; }) .catch(error => console.error('Error:', error));
The Fetch API simplifies the process of making network requests. In this example, data fetched from ‘https://api.example.com/data’ is used to update an element with the ID ‘output’, showcasing a clean and engaging method of handling asynchronous data fetching.
These examples illustrate the diverse applications of JavaScript, highlighting its role in enhancing web page interactivity and user experience. From form validation and dynamic content updates to event handling and asynchronous operations, JavaScript proves to be indispensable in the modern web development landscape.
Advanced JavaScript: ES6 and Beyond
With the advent of ECMAScript 6 (ES6), JavaScript experienced a significant evolution, introducing a plethora of advanced features that have since become indispensable for developers. These enhancements have streamlined code syntax, improved readability, and fortified the language’s capabilities. Notable among these features are let
and const
, arrow functions, template literals, destructuring, and spread/rest operators.
let and const
The let
and const
keywords offer block-scoped variable declarations, which is a significant improvement over the function-scoped var
. The let
keyword allows for mutable variables, while const
is used for immutable variables, preventing reassignment.
let
example:
let name = "John";name = "Doe";
const
example:
const pi = 3.14159;
Arrow Functions
Arrow functions provide a concise syntax for writing function expressions. They also lexically bind the this
value, eliminating common confusion caused by traditional functions.
Example:
const add = (a, b) => a + b;
Template Literals
Template literals offer a more readable and efficient way to create multi-line strings and perform string interpolation using backticks (`
) instead of quotes.
Example:
const greeting = `Hello, ${name}!`;
Destructuring
Destructuring is a convenient way of extracting values from arrays or objects into distinct variables, making the code cleaner and more readable.
Example:
const [x, y] = [1, 2];const {name, age} = {name: "John", age: 30};
Spread/Rest Operators
The spread operator (...
) enables the expansion of an array or object into individual elements. Conversely, the rest operator collects all remaining elements into an array.
Spread example:
const numbers = [1, 2, 3];const newNumbers = [...numbers, 4, 5];
Rest example:
const [first, ...rest] = [1, 2, 3, 4];
Overall, the advanced features introduced in ES6 and beyond have significantly enhanced JavaScript’s power and usability, simplifying complex code structures and improving overall efficiency.
JavaScript Frameworks and Libraries
JavaScript frameworks and libraries play an essential role in modern web development, providing developers with the tools necessary to build sophisticated, feature-rich applications with increased efficiency and reliability. By offering predefined structures and functionalities, they streamline the development process, enabling quicker prototyping, easier maintenance, and enhanced scalability. Let us delve into some of the most popular JavaScript frameworks and libraries, exploring their unique features and typical use cases.
React
Developed by Facebook, React is a highly versatile library for building user interfaces, particularly single-page applications where performance and responsiveness are paramount. React employs a component-based architecture, promoting reusability and manageability of code. Through its virtual DOM, React ensures fast rendering by synchronizing state changes efficiently. React is especially beneficial in scenarios requiring dynamic, real-time user experiences.
Angular
Angular is a comprehensive framework maintained by Google, offering an extensive set of tools for crafting robust, dynamic web applications. Featuring two-way data binding, dependency injection, and a modular approach, Angular excels in building large-scale, enterprise-level applications. Its CLI tools and detailed documentation further enhance productivity, making it a preferred choice for complex, data-intensive projects.
Vue
Vue is a progressive framework praised for its simplicity and ease of integration with existing projects. Created by Evan You, Vue offers a gentle learning curve, making it accessible to newcomers while still providing powerful tools for experienced developers. Its component-based architecture, reactive data binding, and flexibility to scale from a library to a full-fledged framework make Vue adaptable for projects of various sizes and complexities.
jQuery
Though it has been somewhat overshadowed by more modern frameworks, jQuery remains a valuable tool for manipulating the DOM and handling client-side events with a straightforward syntax. It simplifies tasks such as HTML document traversal, event handling, and animation, making it ideal for enhancing legacy systems or projects with specific needs for browser compatibility.
In conclusion, selecting the right JavaScript framework or library largely depends on the project scope, scalability requirements, and the team’s familiarity with the tool. While React, Angular, Vue, and jQuery are among the most popular choices, the landscape of JavaScript tools is vast and continually evolving, offering a variety of solutions tailored to diverse development challenges.
Debugging and Testing JavaScript Code
Debugging and testing are crucial phases in the JavaScript development lifecycle, playing a significant role in ensuring the reliability and functionality of the code. Efficient debugging and testing not only help in identifying and resolving issues but also contribute to the development of maintainable, high-quality, and bug-free applications.
One of the first steps in debugging JavaScript code is utilizing browser developer tools. Modern browsers offer robust development environments that include various debugging features. For instance, Chrome DevTools provides functionalities such as viewing the DOM structure, monitoring network activity, and inspecting and modifying the code in real-time. With these tools, developers can set breakpoints, enabling them to pause code execution at chosen points, allowing for thorough examination of variable states and flow control.
Another fundamental technique is console logging. By inserting console.log()
statements at critical points in the code, developers can track the flow of execution and inspect the values of variables at different stages. This practice is particularly useful for quick debugging but should be used judiciously to avoid cluttering the console with excessive logs.
In addition to debugging, testing is paramount for verifying that JavaScript code performs as expected. Unit testing is a primary method, focusing on individual components or functions to ensure they work independently and correctly. Frameworks such as Jest and Mocha provide comprehensive tools for writing and running unit tests. Jest, for instance, simplifies the process with its built-in assertions and mocking capabilities, while Mocha offers a flexible, modular approach, often used in conjunction with assertion libraries like Chai.
Implementing testing not only catches bugs early but also aids in refactoring, ensuring that changes don’t introduce new issues. Writing tests encourages developers to consider edge cases and error handling, fostering robust and reliable code. Ultimately, a disciplined approach to debugging and testing elevates the overall quality and sustainability of JavaScript applications, making these practices indispensable in professional development environments.
Best Practices and Common Pitfalls
Writing clean, efficient, and maintainable JavaScript code is essential for ensuring long-term sustainability and performance of web applications. Adhering to coding standards, for instance, can greatly enhance the readability and consistency of your code. Utilize consistent naming conventions, indentation, and braces to create a visually cohesive and understandable codebase. Additionally, taking advantage of code linters and formatters can automatically enforce these standards, minimizing human error.
Another critical practice is avoiding the use of global variables. Excessive reliance on global variables can cause unpredictable behavior due to variable name collisions and unintended modifications across different parts of your application. Instead, encapsulate variables within functions or use block-level scope via let
and const
declarations introduced in ECMAScript 6 (ES6).
Modern syntax provides several features that not only make your JavaScript code more concise but also enhance its functionality. Arrow functions, template literals, and destructuring assignments are examples of such enhancements. Embracing these features can streamline your code and improve its readability. Furthermore, the use of modules allows for better organization of your JavaScript code by breaking it down into smaller, reusable components. This modular approach facilitates ease of maintenance and scalability.
Effective error handling is another cornerstone of robust JavaScript programming. Employing try-catch
blocks to manage exceptions can prevent your application from crashing and provide informative error messages to users. Additionally, using tools and libraries like ESLint and Jest can help identify potential errors early in the development process.
Developers also need to be aware of common pitfalls to avoid. One frequent mistake is the mishandling of asynchronous operations, which can lead to issues such as callback hell or unhandled promise rejections. Utilizing Promises and async/await can simplify asynchronous code and enhance its maintainability. Another common issue is misunderstanding the behavior of the this
keyword, particularly in nested functions or callbacks. Properly binding this
within these contexts can circumvent many related issues.
Debugging remains a crucial skill for JavaScript developers. Utilizing the browser’s developer tools, setting breakpoints, and logging values can help trace and fix issues efficiently. Understanding stack traces and leveraging tools like Visual Studio Code Debugger can also enhance the debugging process.