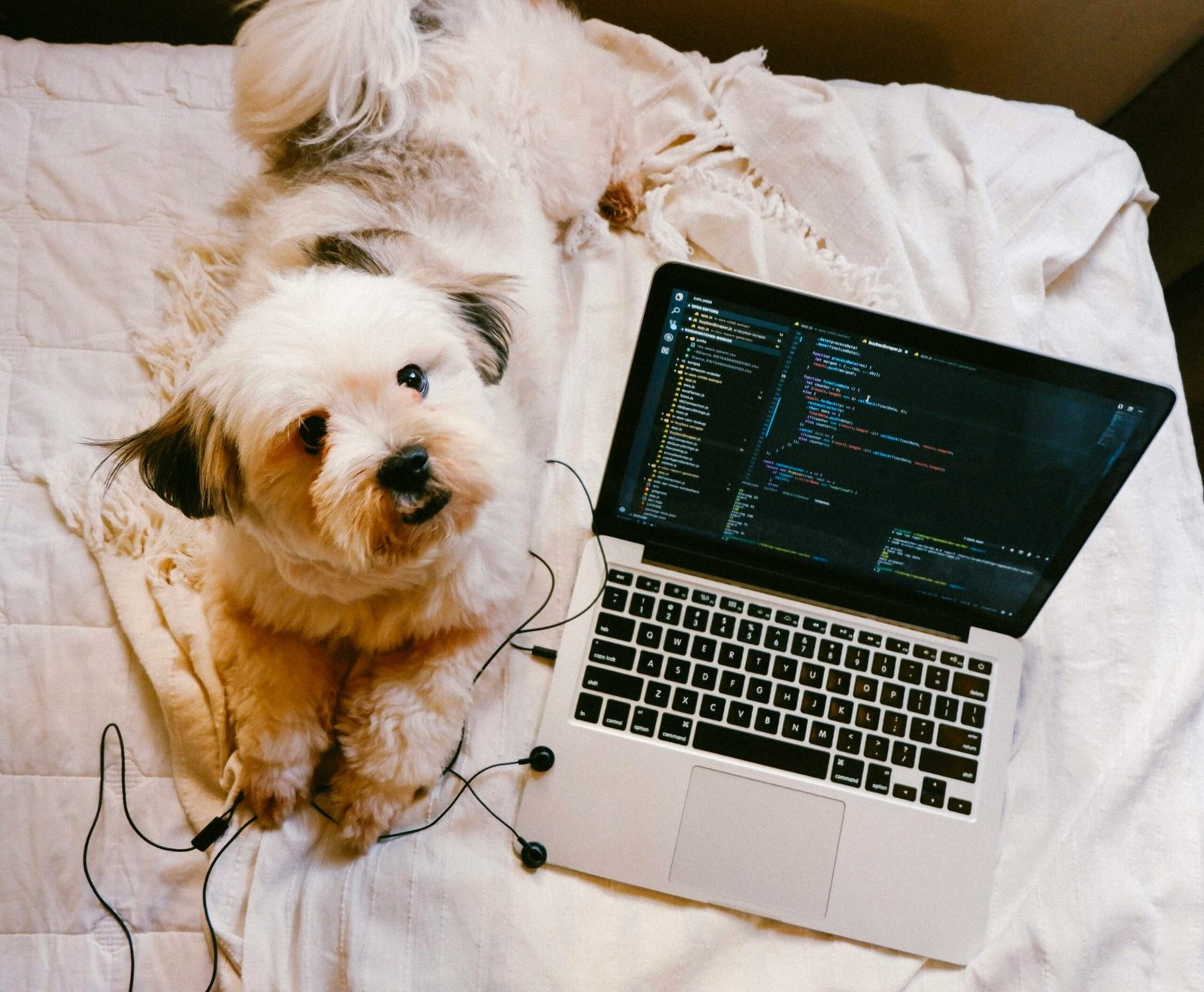
Introduction to Node.js
Node.js is a powerful, open-source JavaScript runtime environment that allows developers to execute code outside of a web browser. Originating in 2009, Node.js was created by Ryan Dahl, revolutionizing the way developers approached server-side programming. The primary motivation behind its development was to address the inadequacies present in existing technologies for handling Input/Output (I/O) operations, particularly in web applications.
Before Node.js, developers often struggled with issues like inefficient I/O operations and limited concurrent connections. Traditional web servers, like those built using Apache, were unable to efficiently handle multiple simultaneous connections, leading to a bottleneck effect. Ryan Dahl sought to overcome these constraints by leveraging the inherent strengths of JavaScript and adapting it for server-side functionalities.
One of the key problems Node.js aims to solve is the blocking I/O inherent in many server environments. Traditional environments operate synchronously, meaning a server must complete one operation before starting another, often resulting in delayed response times and sluggish performance. In contrast, Node.js embraces a non-blocking, event-driven architecture that enables asynchronous I/O operations. This allows the server to handle multiple requests concurrently, vastly improving efficiency and scalability.
Node.js is built on the V8 JavaScript engine, developed by Google for its Chrome browser. This engine compiles JavaScript directly to machine code, offering fast execution times. By utilizing the V8 engine, Node.js harnesses the lightweight and high-performance characteristics of JavaScript, making it ideal for real-time applications, such as chat applications, online gaming, and live streaming.
In essence, Node.js emerged as a transformative solution to the challenges posed by traditional server environments. Its innovative approach to non-blocking, asynchronous I/O has established it as a cornerstone technology for modern web development, enabling developers to build scalable and high-performance applications effortlessly.
Key Features of Node.js
Node.js has become one of the most popular platforms for building scalable and efficient network applications, largely due to its unique set of features. One of the core characteristics of Node.js is its asynchronous and event-driven architecture. Unlike traditional models that execute in a linear, blocking manner, Node.js operates on a single thread using non-blocking I/O calls. This allows it to handle multiple operations simultaneously, making the system exceptionally efficient and fast.
Another significant feature is the V8 JavaScript engine. Developed by Google for its Chrome browser, the V8 engine interprets JavaScript into machine code at a breakneck pace. This enables Node.js to execute scripts with remarkable speed and efficiency, which is especially beneficial for server-side applications that require rapid processing and minimal latency.
Non-blocking I/O is a fundamental aspect that sets Node.js apart. It utilizes an event loop to perform I/O operations – such as reading and writing to file systems, network connections, or databases – in a non-blocking manner. This design ensures that the system remains responsive, allowing it to manage numerous connections and services without being bogged down by I/O operations.
The simplicity in building scalable network applications is another hallmark of Node.js. Its modular architecture and the use of JavaScript make it easier for developers to write, maintain, and scale network applications. Whether you are handling simple HTTP requests or developing complex real-time applications like chat servers, Node.js provides the tools and framework necessary for scalable development.
Finally, the vibrant and active community support adds tremendous value to Node.js. With an ever-growing ecosystem of open-source libraries, modules, and tools, developers can leverage collective knowledge and contributions to enhance their applications. This community-driven approach ensures that Node.js remains current with emerging trends and technologies, fostering innovation and continuous improvement.
The Node.js Architecture
Node.js employs a unique architecture based on a single-threaded model with event looping, distinguishing it from traditional multi-threaded server models. This approach allows Node.js to handle multiple concurrent requests with remarkable efficiency, a crucial advantage in modern web development.
In a traditional multi-threaded request-response model, each incoming request initiates a new thread, potentially leading to significant overhead and resource consumption as the number of requests increases. This design can be efficient for CPU-intensive tasks, but it often struggles under heavy load due to the sheer number of threads it must manage concurrently.
By contrast, Node.js adopts a single-threaded event-driven architecture. Rather than spawning new threads for each request, Node.js uses a single thread to handle all incoming requests through an event loop. This event loop monitors asynchronous operations—such as file I/O, network requests, or database queries—and, upon their completion, triggers callbacks to handle the next steps. The use of non-blocking I/O operations ensures that the main thread remains free to process additional incoming requests without delay.
In this model, tasks that would traditionally block the execution of other operations are instead offloaded to worker threads managed by the underlying operating system or Node.js’s own worker pool. These tasks operate in the background, allowing the main thread to continue event processing unimpeded. The result is a highly scalable architecture capable of handling a vast number of simultaneous connections with minimal performance degradation.
In summary, Node.js’s single-threaded model with event looping excels in handling concurrent connections efficiently. By avoiding the pitfalls of traditional multi-threaded models, it delivers superior performance, particularly in I/O-bound and real-time applications. This innovative architecture is foundational to Node.js’s growing popularity and its adoption in a variety of web and network-oriented applications.
Setting Up Node.js Environment
Setting up a Node.js development environment is a crucial first step for developers looking to leverage its capabilities. The process begins with installing Node.js and npm (Node Package Manager), tools integral to creating and managing Node.js applications. This guide outlines the steps for installing these tools on various operating systems, verifying successful installation, and initializing a basic project.
For Windows users, the easiest route is to download the Node.js installer from the official website. After downloading, run the installer and follow the prompts to complete the installation. By default, this process will also install npm. To verify the installation, open the Command Prompt and execute the commands node -v
and npm -v
; this will display the installed versions of Node.js and npm respectively.
On macOS, developers can use Homebrew, a popular package manager. First, install Homebrew if it is not already installed by executing the command /bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
in the Terminal. Once Homebrew is set up, install Node.js and npm by running brew install node
. Again, verify the installation with node -v
and npm -v
.
Linux users can install Node.js and npm using a package manager like apt or yum. For Debian-based distributions like Ubuntu, the commands are sudo apt update
followed by sudo apt install nodejs npm
. To verify installation, use node -v
and npm -v
as with other operating systems.
Once Node.js and npm are installed and verified, setting up a basic Node.js project is straightforward. Begin by creating a new directory for the project, navigate into it, and run npm init
. This command initializes a new npm project and creates a package.json
file, which will store metadata and dependencies for the project. It prompts for project details like name, version, and entry point, allowing defaults by pressing enter.
By following these steps, developers ensure they have a robust Node.js environment ready to dive into the development process, leveraging the powerful JavaScript runtime to build scalable and efficient applications.
Building Your First Node.js Application
To embark on your journey with Node.js, a practical starting point is the creation of a straightforward “Hello World” application. Follow these step-by-step instructions to set up your first Node.js project.
Begin by ensuring Node.js is installed on your system. You can confirm the installation by running node -v
in your terminal, which should display the current version of Node.js. With Node.js installed, proceed by creating a new directory for your project:
mkdir my-first-node-app && cd my-first-node-app
Next, initialize a new Node.js project within this directory by executing:
npm init -y
This command creates a package.json file with default settings, formally establishing your project. Now, create a new file named app.js which will house your application code:
touch app.js
Open app.js in your preferred text editor and input the following code:
const http = require('http');const hostname = '127.0.0.1';const port = 3000;const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello World\n');});server.listen(port, hostname, () => { console.log(`Server running at http://${hostname}:${port}/`);});
This code imports the HTTP module, sets the server configurations, and defines a simple server that responds with “Hello World” to any incoming requests. Next, proceed to run your server by executing:
node app.js
If everything is set up correctly, you will see a message indicating that the server is running:
Server running at http://127.0.0.1:3000/
Open your web browser and navigate to http://127.0.0.1:3000/
. You should see the “Hello World” message displayed on the screen. Congratulations! You have successfully created and run your first Node.js application.
This basic “Hello World” application lays the foundation for more complex projects, illustrating the simplicity and efficiency of building web servers with Node.js. As you continue to explore Node.js, you will discover its powerful capabilities and extensive ecosystem.
Core Modules and NPM
Node.js, as a robust and flexible runtime environment, provides a comprehensive set of core modules to enhance development efficiency. These core modules are preloaded libraries offering essential functionalities without necessitating external dependencies. One such module is the http
module, pivotal for creating and handling server functionalities, allowing developers to set up web servers and manage requests and responses seamlessly. Another critical module is the fs
(File System) module, which provides an API to interact with the file system, making it straightforward to read, write, and manipulate files and directories.
The path
module simplifies working with file and directory paths, ensuring smooth path manipulations across different operating systems. Meanwhile, the os
module offers operating system-related utility methods and properties, providing insights into the operating system’s architecture, total memory, and more. Additionally, the util
module serves as a collection of utility functions, facilitating tasks like formatting strings and debugging.
Apart from core modules, Node.js leverages the power of npm (Node Package Manager), the largest software registry available in the JavaScript ecosystem. npm simplifies the process of installing, updating, and managing packages and dependencies, streamlining development workflows. To install a package, developers can use the command npm install package-name
, adding functionality swiftly to their projects. Managing project dependencies is made easy with npm, where the package.json
file captures and maintains all necessary details, ensuring consistency and reliability across different environments.
Several popular npm packages significantly enhance Node.js development. Express, for instance, is a minimalist web framework streamlining server and API development with a lightweight structure. Lodash, a utility library, offers a plethora of functions for manipulating and traversing data structures, greatly improving coding efficiency. Mongoose, an essential ODM (Object Data Modeling) library for MongoDB, enables seamless interaction with MongoDB databases, making data modeling and validation straightforward.
Handling Asynchronous Operations
Node.js, being event-driven, excels in handling asynchronous operations, which are crucial for non-blocking I/O tasks. Three primary techniques to manage asynchronous operations in Node.js are callbacks, promises, and async/await. Each method offers unique advantages and is suitable for different scenarios, enhancing the efficiency and readability of the code.
Callbacks are the traditional way of handling asynchronous operations in Node.js. A callback function is passed as an argument to another function and is executed after the completion of an asynchronous task. Although effective, callbacks can lead to deeply nested, hard-to-read code known as “callback hell,” making maintenance challenging. However, callbacks still have their place in simple, linear asynchronous tasks.
Promises provide a more modern approach to managing asynchronous operations, addressing the drawbacks of callbacks. A promise represents a value that may be available now, later, or never. They allow chaining operations using “.then()” and “.catch()”, providing a cleaner, more manageable syntax than callbacks. Promises help reduce code nesting and enhance error handling, gaining popularity in contemporary Node.js applications.
Async/await, built on promises, offers the most elegant way to handle asynchronous operations, making asynchronous code appear synchronous. By using the “async” keyword before a function and “await” for promises, developers can write more readable, straightforward code. Error handling becomes more intuitive using “try” and “catch” blocks, making async/await the preferred choice for complex asynchronous applications.
Best practices for handling asynchronous operations in Node.js include using callbacks for simple asynchronous tasks, promises for more structured and chained operations, and async/await for the most readable and maintainable code. Choosing the appropriate method depends on the complexity and requirements of the task at hand. By understanding and leveraging these techniques, developers can improve the performance and clarity of their Node.js applications.
Building a RESTful API with Node.js
Building a RESTful API with Node.js offers a powerful yet flexible approach to backend development. By leveraging Express, a minimalist web framework for Node.js, you can streamline server creation, route definition, and request handling. Start by setting up your Node.js environment and installing necessary dependencies: Express for route handling, and a database library like Mongoose for MongoDB connectivity.
First, initialize your project with `npm init` and install Express using `npm install express`. To set up the server, create an `app.js` file. Within this file, require Express, initialize the app, and define your server’s entry point:
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
Next, establish routes to manage CRUD operations. For illustration, let’s say we are dealing with a `users` resource. Define routes to handle reading, creating, updating, and deleting user data:
app.get('/users', (req, res) => {
// Retrieve users from database
res.send('List of users');
});
app.post('/users', (req, res) => {
// Add new user to database
res.send('User created');
});
app.put('/users/:id', (req, res) => {
// Update existing user
res.send('User updated');
});
app.delete('/users/:id', (req, res) => {
// Delete user from database
res.send('User deleted');
});
Connecting to a database is essential for persisting data. If using MongoDB, install Mongoose with `npm install mongoose`. Define a connection in `app.js`:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/yourdbname', { useNewUrlParser: true });
Create Mongoose models to define schemas for your data, and update route handlers to interact with the database through these models. Implement schema validation to ensure data integrity. Finally, validate your API by testing endpoints using Postman or similar tools. Ensure each route performs the intended operation by handling errors gracefully and returning appropriate status codes.
By following these steps, you can develop a robust RESTful API with Node.js and Express, providing the foundation for scalable and efficient web applications.