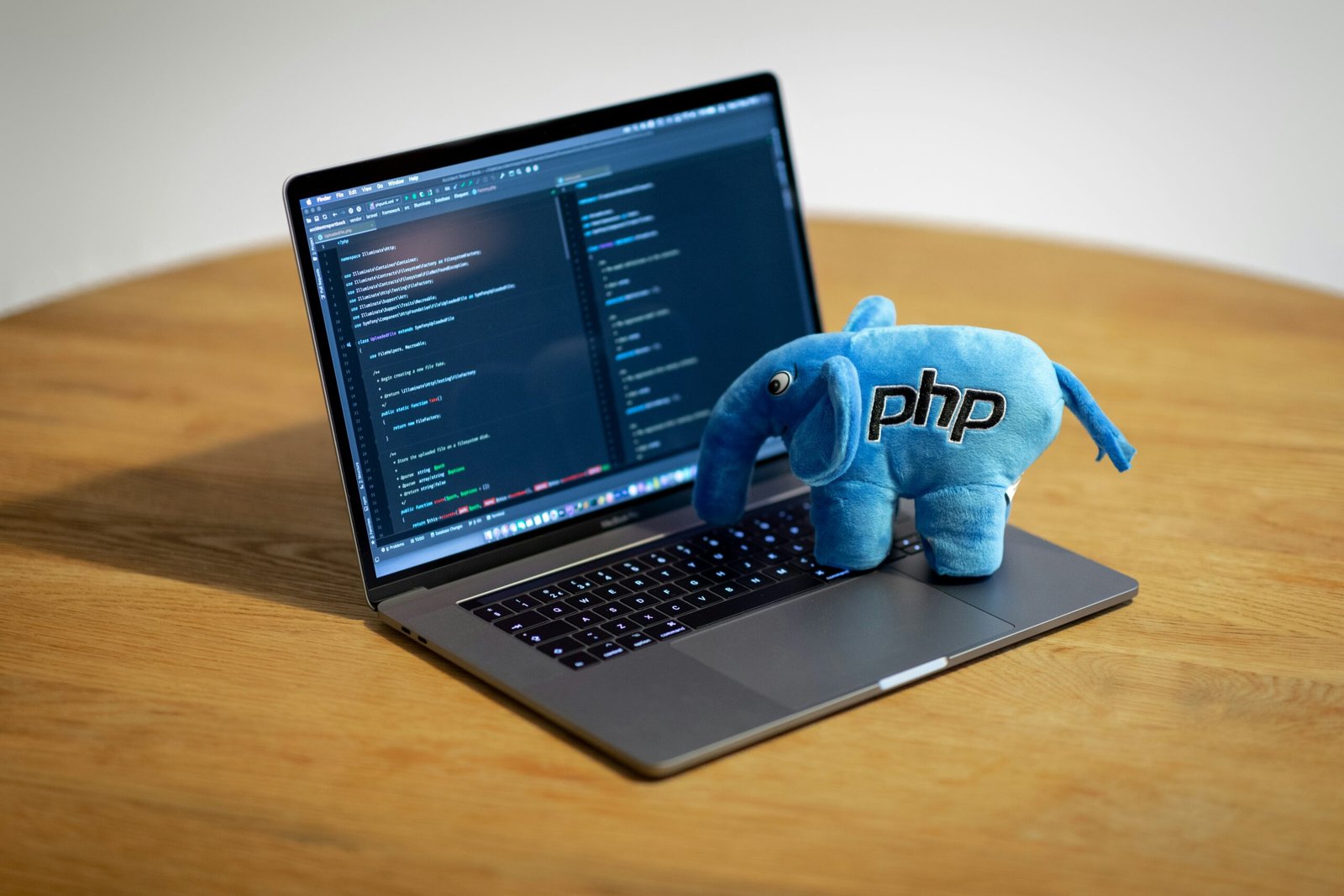
Introduction to PHP
Hypertext Preprocessor, commonly known as PHP, is a widely-used, open-source scripting language that is especially suited for web development. Initially launched in 1995 by Rasmus Lerdorf, PHP has undergone significant evolution, growing from a set of CGI binaries written in C to a fully-fledged programming language. The transformation has been nothing short of remarkable, with PHP consistently adding new features, improved security, and performance enhancements over the years.
PHP’s popularity can largely be attributed to its ease of use and its ability to seamlessly integrate with HTML. This makes it an ideal choice for creating dynamic web pages and server-side scripting. Compatibility with various databases, such as MySQL, PostgreSQL, and SQLite, further enhances its versatility, making PHP a robust solution for a wide array of web applications. Additionally, the language’s extensive library of built-in functions and vast community support contribute to its widespread adoption.
Despite being a language with a rich legacy, PHP continues to play a critical role in modern web development. It powers approximately 78% of websites, including major platforms like WordPress, Facebook, and Wikipedia, as per statistics from W3Techs. PHP’s relevance endures, driven by continuous updates and the introduction of frameworks such as Laravel and Symfony. These frameworks offer developers an array of tools to build scalable, secure, and high-performing web applications rapidly.
In an era where web technologies are rapidly evolving, PHP remains a pivotal tool in a developer’s toolkit. It provides an accessible entry point for learners while offering advanced capabilities for seasoned professionals. The language’s adaptability and consistent improvements ensure that it remains integral to the landscape of web development, addressing both current and future needs effectively.
Setting Up the PHP Environment
Setting up the PHP development environment is a crucial first step for any web developer looking to harness the power of this essential language. To begin with, you need to download and install PHP. The current official PHP distribution is available directly from the [PHP website](https://www.php.net/downloads). Be sure to select the appropriate package for your operating system.
Once PHP is installed, the next step involves setting up a local server. Tools such as XAMPP and MAMP are popular choices due to their ease of use and comprehensive features. XAMPP is an open-source package that includes Apache HTTP Server, MariaDB, and interpreters for PHP and Perl. It’s an excellent choice for Windows, macOS, and Linux users. Alternatively, MAMP is a similar solution particularly favored among macOS users, offering Apache, Nginx, MySQL, and other useful server-oriented tools. Both solutions simplify the process by bundling the essential components needed to run PHP applications locally.
If you prefer a more hands-on approach, you can manually configure your PHP environment. This involves installing a web server like Apache or Nginx and a database server such as MySQL or MariaDB separately, followed by setting up PHP. The manual setup allows for greater customization but can be more complex and time-consuming. Key configuration steps include setting up the PHP.ini file, which allows you to define essential directives and modify default behavior to suit your development needs. Furthermore, ensuring that your system’s PATH variable correctly points to the PHP executable is vital for seamless operation.
Whichever method you choose, ensure your environment is properly configured to support your development workflow. Testing your setup by creating a simple PHP file with the phpinfo()
function will confirm that PHP is correctly installed and running. With the environment up and running, you are now prepared to delve deeper into PHP’s robust functionalities and start building dynamic, data-driven web applications.
PHP Syntax and Basic Constructs
PHP, an acronym for Hypertext Preprocessor, is a widely-utilized open-source scripting language designed especially for web development. Understanding the syntax and basic constructs of PHP is essential for anyone looking to harness its full potential.
At its core, PHP is embedded within HTML. PHP scripts are predominantly executed on the server, and the result is returned to the browser as plain HTML. A PHP script starts with the and ends with the
?>
tags. Within these tags, developers can write PHP code seamlessly mixed with HTML.
Variables are a fundamental part of PHP syntax and are denoted by a leading dollar sign ($
). PHP is a loosely typed language, meaning that a variable’s type does not need to be declared explicitly. Variables can store data types such as strings, integers, floats, booleans, arrays, objects, and resources.
Constants, unlike variables, have immutable values. They are defined using the define()
function and are always written in uppercase letters as a common convention. Operators are used to perform operations on variables and values, and PHP supports a wide range of operators, including arithmetic, assignment, comparison, and logical ones.
Control structures in PHP enable decision-making processes within the script. Conditional statements like if
, else
, and switch
allow the execution of code blocks based on specified conditions. The if
statement is straightforward and executes the block of code if the condition evaluates to true. The else
statement provides an alternative block of code if the condition in if
is false. The switch
statement evaluates a single expression and executes corresponding blocks of code based on the expression’s value.
Loops are indispensable constructs for executing a block of code repeatedly. PHP offers several types of loops: the for
loop, the while
loop, and the do-while
loop. The for
loop is used when the number of iterations is known ahead of time. The while
loop continues to execute as long as its condition remains true. The do-while
loop is similar but ensures the code block is executed at least once before the condition is tested.
Mastering the basic syntax and constructs of PHP forms the foundation for more advanced PHP programming concepts. Aspiring PHP developers should prioritize a strong understanding of these fundamentals to ensure they can effectively utilize PHP in web development projects.
Working with Functions
Functions are an integral part of PHP programming, allowing developers to encapsulate code into reusable blocks. PHP provides a vast array of built-in functions that help streamline common tasks, such as string manipulation, date handling, and database interactions. However, user-defined functions enable programmers to create custom logic tailored to specific needs.
Defining a user-defined function in PHP is straightforward. The syntax involves the function
keyword followed by the function name and a pair of parentheses. Inside the parentheses, you can define parameters, which serve as placeholders for the values passed to the function when it is called. A standard function definition looks like this:
function functionName($parameter1, $parameter2) {
// function body
return $result;
}
Passing parameters to functions in PHP allows for greater flexibility. Parameters act as variables that are accessible within the function’s scope. When a function is called, the actual values of these parameters are provided, making the function’s output dynamic. PHP supports both passing parameters by value and by reference. Passing by value means that the function gets a copy of the argument, whereas passing by reference means the function can modify the original variable.
To retrieve a value from a function, the return
statement is used. This keyword not only terminates the execution of the function but also sends back a value to the code that called the function. By leveraging return values, developers can craft functions that process data and then pass results back for further operations.
Variable scope in PHP is another concept crucial to understanding functions. Variables declared within a function are local to that function and cannot be accessed outside it. Conversely, variables declared outside any function have a global scope. PHP provides the global
keyword to access global variables within a function, but it’s generally advisable to minimize global variable usage to avoid unexpected side effects.
Anonymous functions, also known as closures, add another layer of functionality to PHP. These functions, which lack a specified name, can be assigned to variables and even passed as arguments to other functions. Closures are often used in callback and filter functions. Here is an example of an anonymous function:
$greet = function($name) {
return "Hello, $name!";
};
echo $greet("World");
By mastering functions in PHP, developers can write more modular, maintainable, and efficient code. Whether utilizing built-in functions or crafting user-defined ones, the flexibility and power that functions offer make them indispensable in any PHP development project.
Handling Forms and User Input
PHP is a robust language for managing forms and user input, making it indispensable for modern web development. This section delves into how PHP interacts with HTML forms, covering the essential methods of data transmission, validation, and security concerns which are critical when dealing with user-generated content.
Creating HTML forms is the first step. In a basic form, an action attribute specifies the PHP file that will process the form data, while the method attribute determines how the data will be sent. For example, to create a form that sends data using the POST method:
<form action="process.php" method="post">
Name: <input type="text" name="name"><br>
Email: <input type="email" name="email"><br>
<input type="submit" value="Submit">
</form>
The GET and POST methods are pivotal in PHP for form handling. The GET method appends form data to the URL, making it suitable for retrieval actions without sensitive information. Conversely, the POST method sends data securely within the HTTP request body, preferred for transactions and sensitive information.
Once the data is received, validating and sanitizing user input becomes crucial to ensure data integrity and security. PHP offers built-in functions such as `filter_var()` for validation and `htmlspecialchars()` for sanitization. For instance, to validate an email address and sanitize a name:
$name = htmlspecialchars($_POST["name"]);
$email = filter_var($_POST["email"], FILTER_VALIDATE_EMAIL);
To prevent critical security risks like SQL injection and Cross-Site Scripting (XSS) attacks, implementing prepared statements and proper input escaping is imperative. Where SQL injection can breach your database, XSS exploits can hijack user sessions. Using PHP’s PDO (PHP Data Objects) for SQL queries with prepared statements mitigates these risks:
$stmt = $pdo->prepare("INSERT INTO users (name, email) VALUES (:name, :email)");
$stmt->execute([":name" => $name, ":email" => $email]);
By integrating these practices, PHP developers can adeptly manage form data, guaranteeing that user interaction with the web application remains secure, reliable, and efficient.
Connecting to a Database with PHP
In the realm of web development, the capability to interact with databases is crucial, enabling the storage, retrieval, and manipulation of data. PHP, renowned for its flexibility and efficiency, offers robust mechanisms to establish connections with various databases, including MySQL and PostgreSQL. One of the most effective and secure methods for these database interactions is the use of PHP Data Objects (PDO).
PDO represents a consistent interface for accessing databases in PHP, abstracting the database-specific code and allowing developers to switch databases with minimal code alterations. To connect PHP to a MySQL database using PDO, one begins by creating a PDO instance. The constructor of this instance requires specific parameters: the Data Source Name (DSN), the username, and the password. Below is an illustrative example:
try { $dsn = 'mysql:host=your_host;dbname=your_db'; $username = 'your_username'; $password = 'your_password'; $options = array( PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION ); $pdo = new PDO($dsn, $username, $password, $options); echo 'Connection successful!';} catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage();}
This script attempts to connect to the MySQL database, utilizing exception handling to manage potential connection errors. A similar approach can be used for PostgreSQL, modifying the DSN to reflect the appropriate database type and parameters:
try { $dsn = 'pgsql:host=your_host;dbname=your_db'; $username = 'your_username'; $password = 'your_password'; $options = array( PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION ); $pdo = new PDO($dsn, $username, $password, $options); echo 'Connection successful!';} catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage();}
After establishing a connection, executing queries and handling results becomes straightforward. For instance, to execute a SELECT query and fetch results:
$query = "SELECT * FROM your_table";$stmt = $pdo->query($query);$results = $stmt->fetchAll(PDO::FETCH_ASSOC);foreach ($results as $row) { echo $row['column_name'];}
This example demonstrates executing a SELECT query and fetching results as an associative array. These techniques highlight the importance of using PDO for database interactions in PHP, ensuring both security and efficiency in web applications.
Error Handling and Debugging in PHP
In the realm of web development, handling and debugging errors in PHP is critical for creating robust and reliable applications. PHP categorizes errors into three main types: syntax errors, runtime errors, and logical errors.
Syntax errors occur when the code fails to conform to PHP’s syntax rules, resulting in parsing failures. These are typically the easiest to identify and correct, as PHP provides clear error messages indicating the location and nature of the mistake. Runtime errors, on the other hand, manifest during code execution and are often due to unforeseen conditions, such as attempting to access a file that does not exist. Logical errors are the most insidious, as the code runs without producing any overt errors, yet the outcome is incorrect due to flaws in the logic.
PHP offers several built-in functions and error reporting levels to aid in error handling. The error_reporting()
function allows developers to specify which errors should be reported, using constants like E_ERROR
, E_WARNING
, and E_NOTICE
. Employing these appropriately ensures that all relevant issues are surfaced without overwhelming developers with unnecessary information. The set_error_handler()
function provides further control by allowing the creation of custom error handlers that can log errors, display user-friendly messages, or even halt script execution as necessary.
Debugging PHP code is significantly streamlined by tools such as Xdebug, which offers advanced capabilities including stack traces, memory allocation tracking, and real-time code execution feedback. Integrating Xdebug into a development environment allows for interactive debugging sessions where breakpoints can be set, variables inspected, and code execution controlled line-by-line. This detailed level of insight is invaluable for diagnosing and resolving complex issues.
Implementing structured error handling and leveraging powerful debugging tools are key practices in PHP development. These methodologies not only enhance the stability and performance of applications but also significantly reduce the time and effort required to maintain and enhance them.
Advanced PHP Concepts and Best Practices
As developers progress from basic PHP towards more advanced operations, a thorough understanding of object-oriented programming (OOP) becomes crucial. OOP in PHP entails the creation and manipulation of objects, encapsulating data and behavior. This methodology brings robustness and reusability to codebases, easing maintenance and scalability. PHP supports OOP through classes and objects, allowing for inheritance, polymorphism, and encapsulation. These principles let developers build modular and adaptable applications.
Namespaces in PHP serve as a powerful tool for avoiding name collisions in larger projects. By grouping related classes, interfaces, and functions within a named context, namespaces enable developers to manage code more efficiently. This mechanism proves particularly useful when integrating multiple third-party libraries, ensuring seamless coexistence by preventing naming conflicts.
Traits, another advanced feature in PHP, provide a mechanism for code reuse without the limitations of single inheritance. Traits allow developers to horizontally compose behaviors within classes, promoting cleaner and more maintainable code. By incorporating reusable methods from traits, classes benefit from shared functionalities without resorting to complex inheritance hierarchies.
Composer, the de facto dependency manager for PHP, simplifies the management of libraries and frameworks. It automates the process of resolving, installing, and updating dependencies, ensuring consistency across development environments. Leveraging Composer effectively streamlines project setup and reduces the risk of conflicting library versions, enhancing development efficiency.
Adhering to best practices in code organization is essential for maintaining clean and scalable PHP applications. Developers should follow SOLID principles, employ PSR (PHP Standards Recommendations) standards, and structure projects logically. Performance optimization is also paramount; techniques such as opcode caching, database indexing, and content caching markedly improve application responsiveness.
Security best practices are integral to PHP development. Developers must sanitize and validate input, employ prepared statements to mitigate SQL injection risks, and use HTTPS to secure data transmission. Regularly updating dependencies and staying abreast of security patches contribute to safeguarding applications from vulnerabilities.